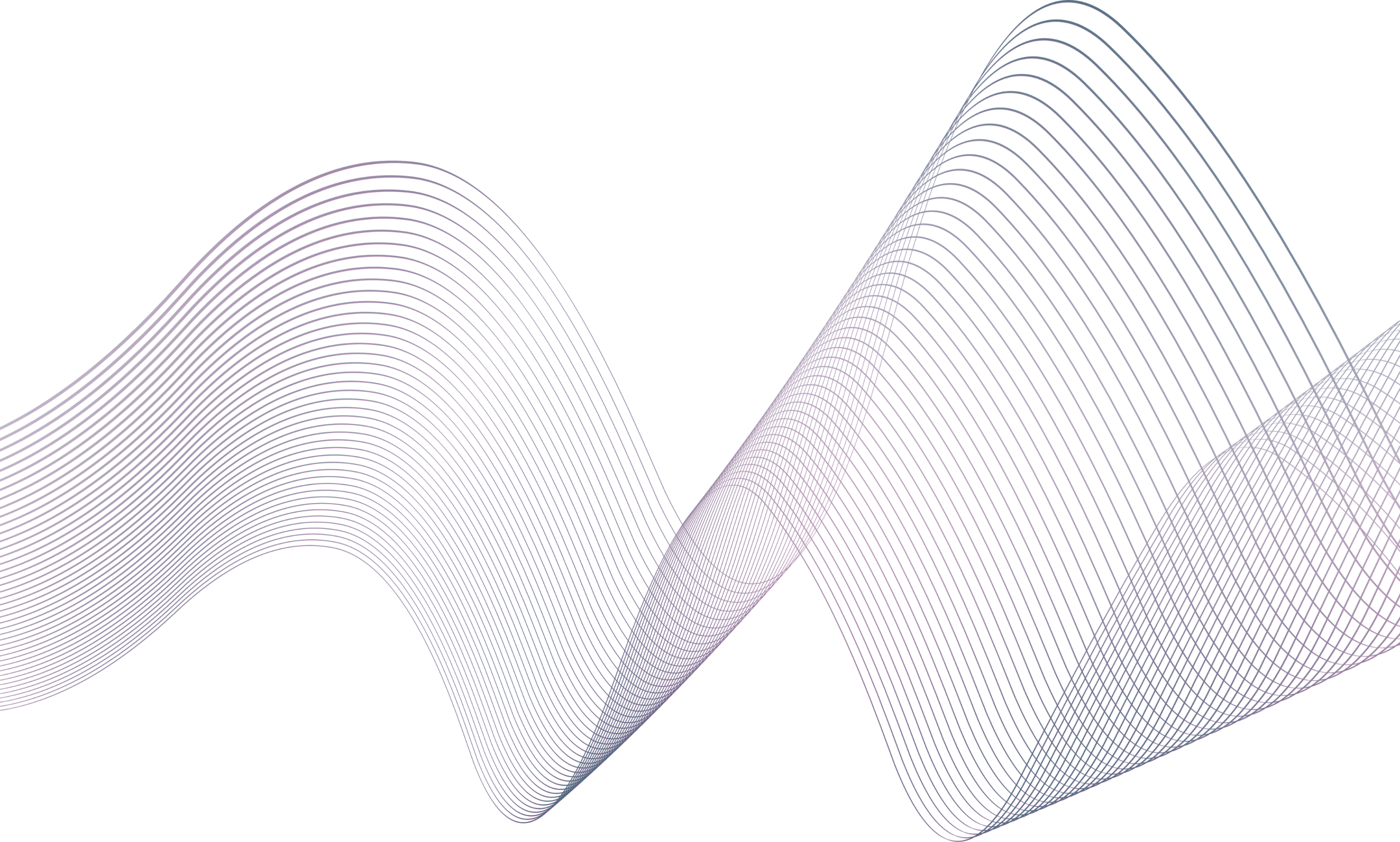
Implementing Microfrontends with React and Next.js
WEB DEVELOPMENT, OPERATIONS.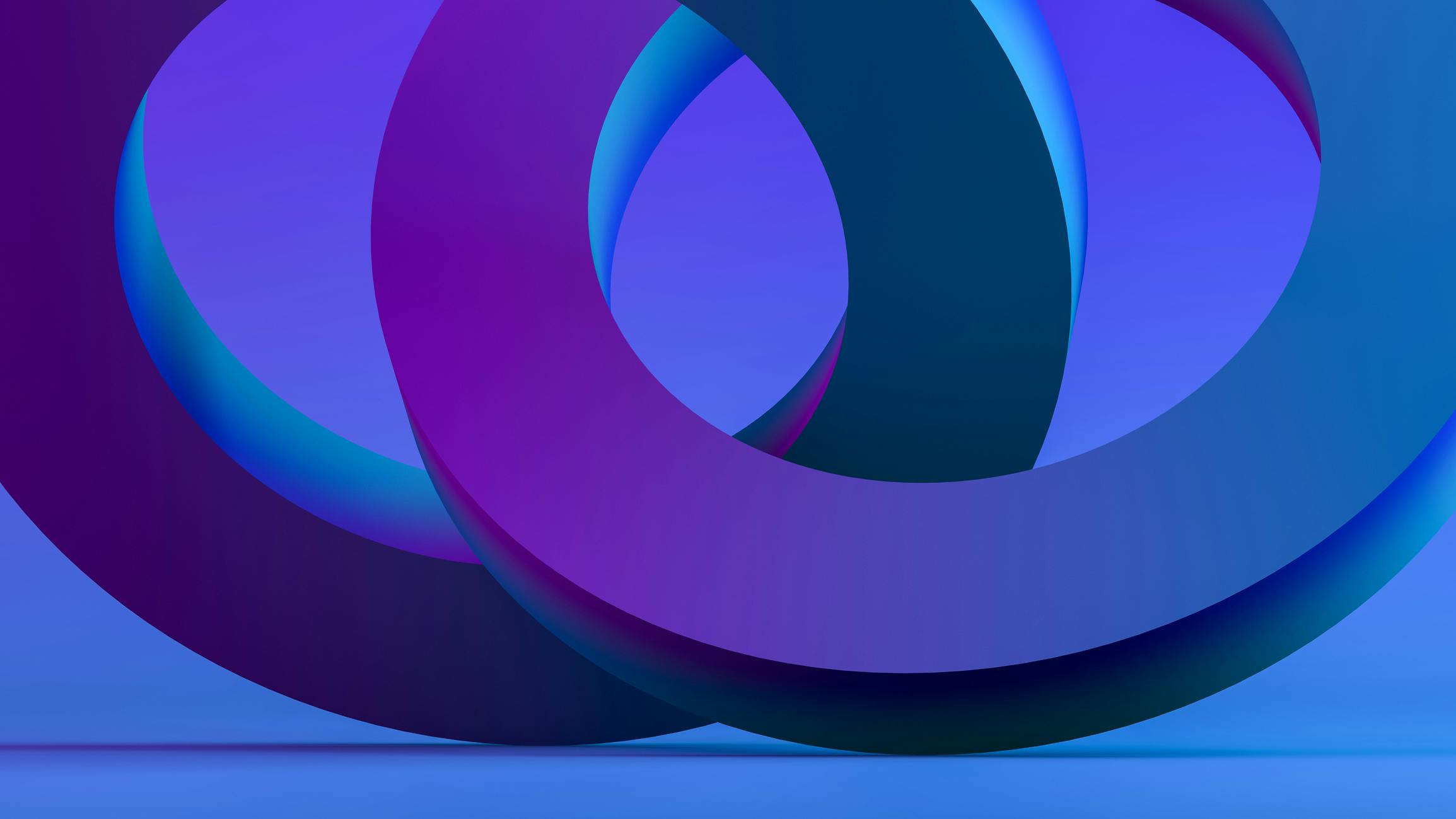
Implementing Microfrontends with React
Implementing Microfrontends with React can offer benefits such as increased modularity and code reuse, improved loading speed and performance, ease of maintenance and updating, flexibility in development, and the ability to work in parallel on different parts of the application.
Reusable component design
By following these steps you can design reusable components that can be used in multiple Microfrontends.
Identify the components that can be reused by determining which ones are common across multiple Microfrontends.
Create a package containing all the components to be reused. This package can be imported into each Microfrontend that needs to use these components.
Use encapsulation to avoid style conflicts.
Use composition to create complex components. This way, they can be customized to fit the needs of each Microfrontend.
.Use propTypes and defaultProps to ensure compatibility and define the properties expected by each component to be compatible with each other.
Use hooks to share logic and avoid code duplication.
Microfrontends Integration
These are the general steps:
Identify the Microfrontends that make up the application. Each must be an independent application and have its own CI/CD repository and pipeline.
Define a communication strategy between the Microfrontends such as events, messages, API calls, among others.
Define the structure of the React application. It can be a folder structure that separates the Microfrontends and their components.
Create a common framework that includes a component structure and a set of rules for component style and interaction.
Implement a container for Microfrontends that can load and unload them, as needed. Technologies such as iFrames, web components or React Portals can be used.
Configure the communication between the Microfrontends through the communication strategy defined above.
Implement navigation between Microfrontends with technologies such as React Router or a custom router.
Test the integration by performing tests on the application to ensure that the Microfrontends communicate correctly and that the navigation works as expected.
Unit and integration testing
By following these steps you can create effective tests that help identify and solve problems.
Select a testing tool available for React such as: Jest, Enzyme, React Testing Library.
Create unit tests for each React component to ensure that it works correctly and meets the specific requirements of the app. Unit tests may include verification of component handling, data and events.
Create integration tests for Microfrontends to test how the different components integrate and work together. These tests may also include verifying that navigation between the different Microfrontends works correctly.
Set up the test environment, ensuring that all necessary packages and dependencies are installed.
Execute tests manually or automatically when changes to the code are detected.
Adjust and improve tests to address bugs and cover more use cases making sure Microfrontends work correctly in all situations.
React Microfrontends Tools and Libraries
Both Module Federation and Single-SPA are powerful tools for building highly modular and scalable Microfrontends applications with React.
Module Federation
It is used to share modules between different applications, so that an application can load components from other applications dynamically and at runtime. It works by creating a federation server that serves as an entry point for sharing modules between different applications.
Single-SPA
It is a Microfrontends library used to create single-page applications (SPAs) composed of multiple independent React applications.
With Single-SPA, each React application runs in its own process and can be dynamically loaded and unloaded as needed.
Challenges of Integrating Microfrontends with React
CSS and JS isolation
There are techniques that can be used to achieve isolation and ensure that applications work consistently and without interference.
1. CSS Modules are used to ensure that CSS styles are isolated within each component, so each React module has its own CSS style file, allowing developers to ensure that they do not mix with each other.
2. Shadow DOM allows developers to encapsulate HTML, CSS and JavaScript within a specific DOM element, so styles and scripts of a React component are kept isolated within an encapsulated DOM "tree", ensuring that they do not affect other components on the page.
3. Loading CSS and JS dynamically so that styles and scripts are loaded only when a specific component is needed, which helps avoid interference with other components.
Dependency resolution
It is important to ensure that applications work properly together. Here are some techniques:
1. Use compatible versions of dependencies. This may require close communication between the development teams of each application to coordinate versions.
2. Use a shared dependency manager such as Yarn or npm. This allows all Microfrontends applications to share the same dependencies and helps avoid version conflicts.
3. Use a demand-driven module loading strategy so that modules are dynamically loaded only when needed.
Scalability and deployment
Here are some options:
1. Use a scalable cloud infrastructure so that Microfrontends applications can easily scale as demand increases. Cloud solutions such as Amazon Web Services (AWS) and Microsoft Azure offer tools and services to do this automatically.
2. Separate business logic and user interface to improve modularity and component reuse.
3. Implement a continuous deployment strategy to reduce errors and improve efficiency. Changes and upgrades can be implemented quickly without service interruptions.
4. Use dynamic routing to allow users to navigate between different applications on a single web page without reloading it. This can improve the user experience and allow for greater flexibility in managing Microfrontends applications.
Implementing Microfrontends with Next.js
Implementing Microfrontends with Next.js can offer benefits such as improved performance and loading speed, easier development and deployment, modularity and code reuse, improved state management, and ease of scaling.
Design of reusable components
Some specific techniques that can be used include:
1. Design components that do not depend on any specific business state or logic. In this way, they can be used in different contexts and applications.
2. Using common design patterns such as facade, container, and presenter can help separate presentation logic from business logic and make components easier to reuse.
3. Establish a centralized component library that can be used across different Microfrontends.
4. Use modular CSS to design components that are independent of the rest of the application. This can prevent the styling of one component from affecting other components or parts of the application.
5. Use automation tools such as Storybook and Styleguidist to help document and test components more efficiently.
Microfrontends and Next.js integration
The integration of Microfrontends and Next.js can be achieved using a variety of approaches. The choice of this will depend on the specific requirements of the application and the needs of the development team.
Use Webpack modules to share and load Microfrontends.
Use API Gateway to route user requests to different Microfrontends based on the requested path. API Gateway can act as a reverse proxy to route the request to the corresponding Microfrontend and return the response to the user.
Iframe-based integration to join different Microfrontends in a Next.js application. Each Microfrontend can be loaded into an iframe and communicate with the Next.js application using postMessage.
Custom event-based integration. Events can be used to share data and actions between the Microfrontends and the Next.js application.
Use the Composite design pattern. Each Microfrontend can be designed as an independent component that can be dynamically added and removed as needed.
Unit and integration testing
The following steps can be taken to perform unit and integration testing:
1. create unit tests for each Microfrontend to ensure that it works properly before integrating it with the Next.js application. Unit tests can test individual components, functions, and any other business logic within the Microfrontend.
2. Create integration tests for the Next.js application to test the full functionality of the application, including all Microfrontends. These can test navigation, user functionality, and integration.
3. Use testing tools such as Jest, React Testing Library, Cypress and Puppeteer.
4. Use mocks and stubs to simulate the behavior of components or modules that are not present in the test application. This can help simplify the process and reduce dependency on other systems.
5. Use separate test environments to avoid affecting the performance and stability of the production application.
Microfrontends Tools and Libraries with Next.js
Module Federation and Single SPA can also be used to create applications composed of several Microfrontends developed with Next.js.
Module Federation allows sharing modules between different applications, which can help reduce package size and speed up application loading time and Single SPA can be used to create a main application consisting of several independent Microfrontends developed with Next.js.
Challenges and Considerations of Integrating Microfrontends with Next.js
CSS and JS isolation
For CSS isolation, Next.js uses CSS Modules by default. CSS Modules allow styles to be defined locally and applied only to the specific components that require them.
This helps prevent styles defined in one Microfrontend from affecting others in the composite application. In addition, Next.js also allows you to use Scoped CSS, which is a technique that uses a unique HTML attribute to ensure that styles are only applied to components that have it.
For JS isolation it is important that each Microfrontend has its own context and does not affect others. To do this, Webpack and Module Federation can be used to separate and share JS modules independently between each Microfrontend. This helps ensure that each can be developed and deployed independently without affecting the others.
These practices help avoid conflicts and ensure that each Microfrontend can be developed and deployed independently.
Dependency resolution
In order to achieve a proper dependency resolution, the following steps can be taken:
Identify the dependencies of each Microfrontend through the package.json file.
Manage the shared dependencies to use tools such as Yarn Workspaces to manage them and avoid conflicts.
Use Module Federation to share modules between different applications, reduce package size and speed up application load time. In addition, Module Federation also allows you to manage dependencies automatically and resolve version conflicts.
Establish a continuous integration (CI/CD) process to detect and resolve dependency issues efficiently. This can be achieved using CI/CD tools such as Jenkins, CircleCI or Travis CI.
Scalability and deployment
The following are the steps required to achieve adequate scalability and deployment:
define a deployment strategy that allows deploying Microfrontends independently. This can be done using containerization tools such as Docker or Kubernetes, which allow the application to be packaged in a container and deployed in different environments.
Use a load balancer to distribute the application traffic between different Microfrontends and ensure that the load is distributed in a balanced manner. This helps to ensure application scalability and avoid bottlenecks.
Scale horizontally instead of increasing the resources of a single server, to handle a higher volume of traffic and improve application availability.
Using caching techniques such as CDN (Content Delivery Network) or Varnish can help reduce the load on servers and speed up application load time.
Establishing a continuous integration and continuous delivery (CI/CD) process allows automating the building, testing and deployment of the application to ensure that each Microfrontend is deployed independently and that the composite application is always up to date.
Microfrontends and Aplyca
We are specialists in cloud technology development and consulting, and we help you create optimal digital experiences for your customers. We invite you to learn about our success stories and contact us to discuss your project needs.